/**
* 汽车父类
* @author:凌_惜
* @date:2016年11月4日 上午10:03:49
*/
public class Car {
private String name; //名称
private double price; //租金
private int capacity; //容量
private double weight; //载重
public Car() {
}
public Car(String name, double price, int capacity) {
this.name = name;
this.price = price;
this.capacity = capacity;
}
public Car(String name, double price, double weight) {
this.name = name;
this.price = price;
this.weight = weight;
}
public Car(String name, double price, int capacity, double weight) {
this.name = name;
this.price = price;
this.capacity = capacity;
this.weight = weight;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public int getCapacity() {
return capacity;
}
public void setCapacity(int capacity) {
this.capacity = capacity;
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
}
/**
* 奥迪
* @author:凌_惜
* @date:2016年11月4日 上午10:08:04
*/
public class Aodi extends Car {
public Aodi(String name, double price, int capacity) {
super(name, price, capacity);
}
}
/**
* 马自达
* @author:凌_惜
* @date:2016年11月4日 上午10:10:24
*/
public class Mazida extends Car {
public Mazida(String name, double price, int capacity) {
super(name, price, capacity);
}
}
/**
* 皮卡雪
* @author:凌_惜
* @date:2016年11月4日 上午10:11:11
*/
public class Pikaxue extends Car {
public Pikaxue(String name, double price, int capacity, double goods) {
super(name, price, capacity, goods);
}
}
/**
* 金龙
* @author:凌_惜
* @date:2016年11月4日 上午10:11:55
*/
public class Jinlong extends Car {
public Jinlong(String name, double price, int capacity) {
super(name, price, capacity);
}
}
/**
* 松花江
* @author:凌_惜
* @date:2016年11月4日 上午10:17:50
*/
public class Songhuajiang extends Car {
public Songhuajiang(String name, double price, double goods) {
super(name, price, goods);
}
}
/**
* 依维柯
* @author:凌_惜
* @date:2016年11月4日 上午10:21:39
*/
public class Yiweike extends Car {
public Yiweike(String name, double price, double goods) {
super(name, price, goods);
}
}
/**
* 测试类
* @author:凌_惜
* @date:2016年11月4日 上午10:22:57
*/
public class CarTest {
public static void main(String[] args) {
Car a1 = new Aodi("奥迪A4",500,4);
Car a2 = new Mazida("马自达6",400,4);
Car a3 = new Pikaxue("皮卡雪6",450,4,2);
Car a4 = new Jinlong("金龙",800,20);
Car a5 = new Songhuajiang("松花江",400,4);
Car a6 = new Yiweike("依维柯",1000,20);
System.out.println("==========欢迎使用答答租车系统==========");
System.out.println("您是否要租车:0.是 1.否");
Scanner scan = new Scanner(System.in);
int flag = scan.nextInt();
if (flag == 1) {
System.out.println("==========欢迎下次再来==========");
return;
}
System.out.println("==========您可租车的类型及其价目表==========");
System.out.println("序号\t汽车名称\t\t租金\t\t容量");
System.out.println("1.\t"+a1.getName()+"\t\t"+a1.getPrice()+"元/天\t载人:"+a1.getCapacity()+"人");
System.out.println("2.\t"+a2.getName()+"\t\t"+a2.getPrice()+"元/天\t载人:"+a2.getCapacity()+"人");
System.out.println("3.\t"+a3.getName()+"\t\t"+a3.getPrice()+"元/天\t载人:"+a3.getCapacity()+"人,载货:"+a3.getWeight()+"吨");
System.out.println("4.\t"+a4.getName()+"\t\t"+a4.getPrice()+"元/天\t载人:"+a4.getCapacity()+"人");
System.out.println("5.\t"+a5.getName()+"\t\t"+a5.getPrice()+"元/天\t载货:"+a5.getWeight()+"吨");
System.out.println("6.\t"+a6.getName()+"\t\t"+a6.getPrice()+"元/天\t载货:"+a6.getWeight()+"吨");
System.out.println("==========请输入您要租车的数量:===========");
int flag2 = scan.nextInt();
double totalPrice = 0; //总价格
int totalCapacity = 0; //总容量
double totalWeight = 0; //总载货量
String[] arr = new String[6];
for (int i = 1; i <= flag2; i++) {
System.out.println("请输入第"+i+"辆车的序号:");
int flag3 = scan.nextInt();
switch (flag3) {
case 1:
totalPrice += a1.getPrice();
totalCapacity += a1.getCapacity();
arr[0] = a1.getName();
break;
case 2:
totalPrice += a2.getPrice();
totalCapacity += a2.getCapacity();
arr[1] = a2.getName();
break;
case 3:
totalPrice += a3.getPrice();
totalCapacity += a3.getCapacity();
totalWeight += a3.getWeight();
arr[2] = a3.getName();
break;
case 4:
totalPrice += a4.getPrice();
totalCapacity += a4.getCapacity();
arr[3] = a4.getName();
break;
case 5:
totalPrice += a5.getPrice();
totalWeight += a5.getWeight();
arr[4] = a5.getName();
break;
case 6:
totalPrice += a6.getPrice();
totalWeight += a6.getWeight();
arr[5] = a6.getName();
break;
}
}
System.out.println("==========请输入您要租车的天数:===========");
int flag4 = scan.nextInt();
totalPrice *= flag4;
System.out.println("**********您的账单:**********");
StringBuilder builder = new StringBuilder();
for (int i = 0; i < arr.length; i++) {
if (arr[i] != null && i <= 3) {
builder.append(arr[i] + "\t");
}
}
if (builder.length() != 0) {
System.out.println("可载人的车有:");
System.out.println(builder + "共载人:" + totalCapacity + "人");
}
StringBuilder builder2 = new StringBuilder();
for (int i = 0; i < arr.length; i++) {
if (arr[i] != null && (i == 2 || i > 3)) {
builder2.append(arr[i] + "\t");
}
}
if (builder2.length() != 0) {
System.out.println("可载货的车有:");
System.out.println(builder2 + "共载货:" + totalWeight + "吨");
}
System.out.println("租车总价格为:" + totalPrice + "元");
scan.close();
}
}
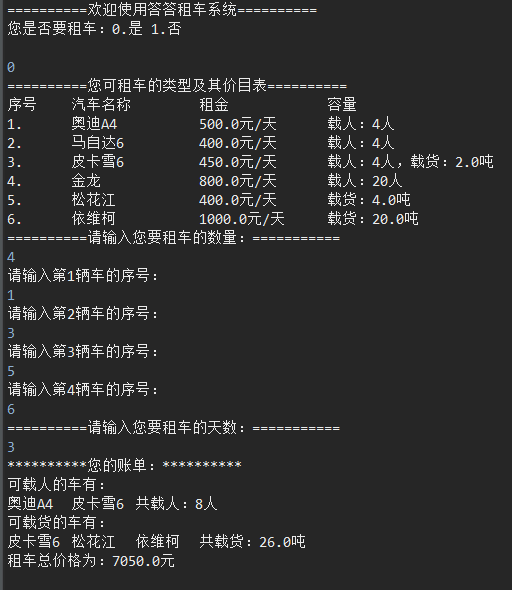
打开App,阅读手记
热门评论
有个疑惑,父类Car中的变量name,price没有用private修饰,也可以用set/get?
我的代码 car类中提示报错。思想和你的一样super,但是提示错我
builder.append是啥意思啊?